Why to use Char Array instead of String for storing password in Java – Security
|Why we should use Character Array for storing sensitive information(like passwords ) instead of Strings in Java is a very important concept with respect to security of the application and this is also one of the favourite questions of any interviewer in any java interview.
So if we try to figure out the difference between these two
What is the biggest difference between a String and a Character Array in Java??
The biggest difference between the two is the way Garbage Collector(GC) handles each of the object. Since Strings are handled by Java Garbage Collector in a different way than the other traditional objects, it makes String less usable to store sensitive information.
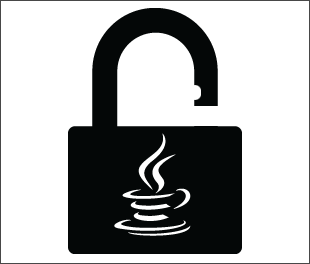
So the main reasons to prefer char[] are-
1) Immutability of Strings
Strings in Java are immutable(i.e. once created we can not change its value) and it also uses the String Pool concept for reusability purpose, hence we are left with no option to clear it from the memory until GC clears it from the memory. Because of this there are great chances that the object created will remain in the memory for a long duration and we can’t even change its value. So anyone having access to the memory dump can easily retrieve the exact password from the memory. For this we can also use the encryption techniques so that if someone access then he will get the encrypted copy of the password.
But with character array you can yourself wipe out the data from the array and there would be no traces of password into the memory.
Read More – What are immutable Strings and what is their benefit.
Have a look at the example and highlighted lines of code.
public class PasswordSecurityExample { public static void main(String[] args) { char[] password = { 'p', 'a', 's', 's', 'w', 'o', 'r', 'd' }; // Changing value of all characters in password for (int i = 0; i < password.length; i++) { password[i] = 'x'; } System.out.print("New Password - "); // Priniting new Password for (int i = 0; i < password.length; i++) { System.out.print(password[i]); } } }
Output:-
New Password - xxxxxxxx
In the above example you can see that the array holding the value of Password is changed and now no traces of the actual password exists in the memory. So anyone even with memory dumps can not retrieve the password.
2) Accidental printing to logs
Along with the memory dump protection storing passwords in Strings also prevent accidental logging of password in Text files, consoles, monitors and other insecure places. But in the same scenario char array is not gonna print a value same as when we use toString() method..
Example:-
public class PasswordSecurityExample { public static void main(String[] args) { String password = "password"; char[] password2; System.out.println("Printing String -> " + password); password2 = password.toCharArray(); System.out.println("Printing Char Array -> " + password2); } }
Output:-
Printing String -> password
Printing Char Array -> [C@21882d18
3) Recommendation by Java itself
Java itself recommends the use of Char Array instead of Strings. It is clear from the JPasswordField of javax.swing as the method public String getText() which returns String is Deprecated from Java 2 and is replaced by public char[] getPassword() which returns Char Array.
Nice explanation. It has always been a topic of debate whether to go for strings or char array for passwords.
I have mentioned few more points at my blog. Please share your thoughts on it.
http://javaterritory.blogspot.com/2014/09/why-use-char-array-for-password.html
@disqus_Oqlg8golKV:disqus .. You are correct that this is really a debatable topic but I had a look at your post and the point you added extra is that arrays are not serializable but this is NOT CORRECT..
Both arrays and Strings are Serializable and neither your point supports the view as even if arrays are not serializable(which is not true) then if we want to store the password we would not be able to which I think suggest you not to use the char array if you want to serialize the class.
@disqus_Oqlg8golKV:disqus You are correct that this is really a debatable topic but I had a look at your post and the point you added extra is that arrays are not serializable but this is NOT CORRECT..
Both arrays and Strings are Serializable and neither your point supports the view as even if arrays are not serializable(which is not true) then if we want to store the password we would not be able to which I think suggest you not to use the char array if you want to serialize the class.
Though the post is off course awesome. Would like to appreciate for your contribution.
Did you think there could be other part of the same question as interviewer some time doesn’t get stop even if you provide correct answer. Please explore more and would recommend to provide reference link also in your discussions. -Thanks
Thank you @disqus_chgpbrbBUS:disqus for your sugestion and kind words.
I will definitely look into it try to putting more depth in the posts. 🙂