BYTE STREAMS in Java – File Reading and Writing Example using Byte Streams
|Performing input and output operations using a Byte Stream is one of the most common and the low level input output operation that a java program can perform. In this data is processed(read/write) in the form of a byte or we can use buffered approach for more structured fast and convenient input output operations.
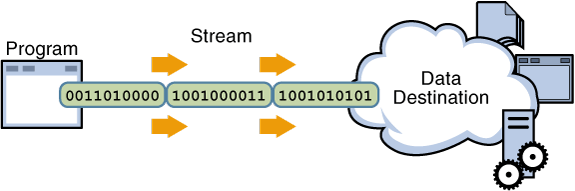
Click here to read Stream basics before continuing with Byte Streams
In Byte Streams all the classes are the descendents of 2 abstract classes-
- InputStream for input operation.
- OutputStream for output operation.
And the 2 most important methods in all the classes that are used for I/O operations are-
- public int read() throws IOException – It reads a byte from Stream.
- public void write(int i) throws IOException – it writes the specified byte into the Stream.
Now lets have a look at Some Important classes for Byte Streams
Stream class | Description |
---|---|
BufferedInputStream | Used for Buffered Input Stream. |
BufferedOutputStream | Used for Buffered Output Stream. |
DataInputStream | Contains method for reading java standard datatype |
DataOutputStream | An output stream that contain method for writing java standard data type |
FileInputStream | Input stream that reads from a file |
FileOutputStream | Output stream that write to a file. |
InputStream | Abstract class that describe stream input. |
OutputStream | Abstract class that describe stream output. |
PrintStream | Output Stream that contain print() and println() method |
File Reading and Writing Example using Byte Streams
- To show you the use of Byte Streams here in this example I will take image as an input file so that it could be clear that Byte Streams can be used for all types of files( except Strings or text files) but ensure that the file is in classpath or provide a fully qualified pathname of the file.
- Also we are using Java 7 feature named try with resources in which we are declaring and Initializing both the Streams along with try and we don’t need to close them explicitly but ensure that every resource you initialize inside try should implement AutoClosable interface.
package codingeekExamples; import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.InputStream; import java.io.OutputStream; public class ByteStreamsExample { public static void main(String[] args) throws IOException { try ( // Declaring and initializing both the Streams inside try so that there // is no need to close them. You can do this with many other classes but // make sure that they all implements the Closable Interface InputStream inputStream = new FileInputStream("input.jpg"); OutputStream outputStream = new FileOutputStream("output.jpg") ) { Integer c; //continue reading till the end of the file while ((c = inputStream.read()) != -1) { //writes to the output Stream outputStream.write(c); } } } }
-> In the beginning I said that this is a low level operation and it should be avoided if you can because this program spends a lot of time in looping i.e reading from input stream and writing to output stream and these operations are really costly.
So what is the need of Byte Streams?
These are of utter importance as all other Streams are basically based upon Byte Stream only and it could be used for all kind of data like images, files, networking operations etc. BUT still there are some cases where we don’t use Byte Streams and prefer to use specialized Streams for that purpose like for reading and writing text files.
Where Byte Streams should not be used?
We should not use Byte Stream for the Streams that represent the character data i.e. for the input output operations of text data. It is because For example if the file uses UNICODE system of encoding than each character will be denoted by two Bytes but Byte Stream will treat them differently and you have to handle the case using some programming logic. Such Streams are known as Character Streams and we use specialized classes for this purpose, Example – BufferedReader, InputStreamReader, FileReader etc.
Resources:- Oracle Byte Streams